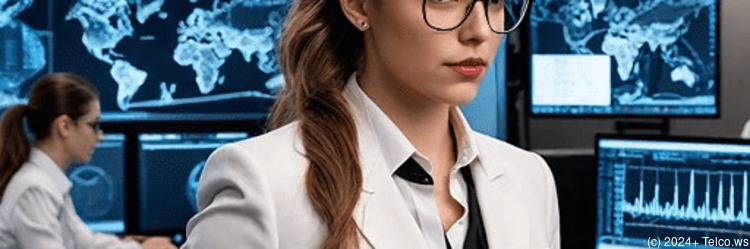
A Comprehensive Look at Design Patterns in Swift Development
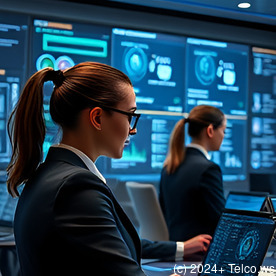
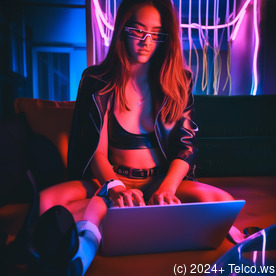
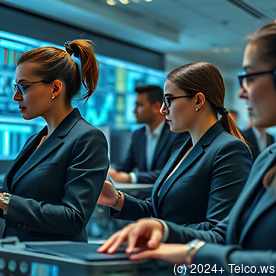
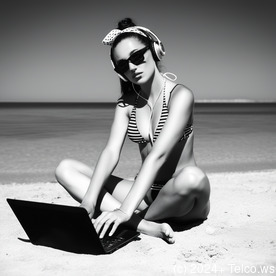
Understanding Design Patterns in Software Development
Design patterns are well-documented solutions to common problems encountered in software design and development. They serve as templates that developers can leverage to address recurring design issues in a flexible and reusable manner. The significance of design patterns in Swift development is profound; they facilitate the creation of applications that are not only maintainable but also scalable. Through the framework offered by design patterns, developers are equipped with a shared vocabulary, which fosters clearer communication of ideas and approaches across teams and projects. This common language is pivotal in collaborative environments where multiple developers work on different parts of the same system.
Furthermore, design patterns encapsulate industry best practices that streamline the development process, significantly reducing the likelihood of errors and bugs that can arise from poorly structured code. They contribute to improved organization, allowing for more effective adaptations to evolving project requirements as developers can rely on established patterns instead of reinventing the wheel. By mastering design patterns, developers can enhance not only their coding skills but also their ability to architect robust, high-quality software that adheres to contemporary industry standards. The learning curve might be steep at first, but the long-term benefits in productivity and efficiency outweigh the initial challenges.
The relevance of design patterns transcends simple coding practices; they embody critical concepts in computer science, enabling developers to identify specific problems and articulate effective solutions. The ability to recognize which design pattern to apply in various situations is crucial for developers aiming to maximize both efficiency and effectiveness in their programming practices. For instance, using the right pattern to delegate responsibilities can prevent feature creep and ensure that applications remain organized even as they grow in complexity.
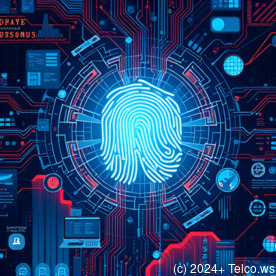
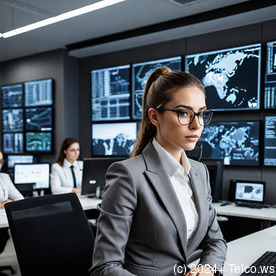
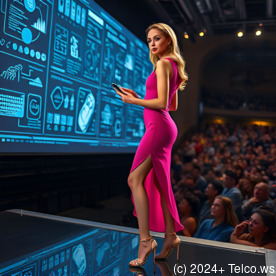
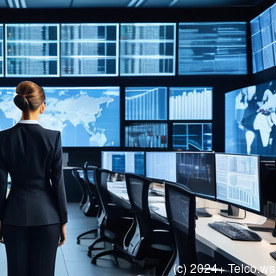
Exploring Design Patterns from Multiple Perspectives
To fully appreciate the role of design patterns in Swift development, its beneficial to evaluate them from a variety of perspectives, which highlights their significance and impact across different contexts.
Economic Benefits
From an economic perspective, adopting design patterns can lead to substantial cost savings in software development. By minimizing debugging time and enhancing code quality, businesses can allocate resources more effectively. Companies that leverage design patterns can experience significant reductions in expenses associated with maintenance and updates. This heightened efficiency results in a higher return on investment, as applications become easier to manage, allowing teams to redirect their focus toward innovation rather than problem-solving. Furthermore, well-designed applications tend to lead to higher customer satisfaction, which in turn increases sales and enhances overall revenue streams.
Historical Context
The historical development of design patterns reflects an evolution in response to the increasing complexity of software engineering. Influential figures such as the Gang of Four, who popularized design patterns in their seminal book "Design Patterns: Elements of Reusable Object-Oriented Software," recognized common pitfalls that developers encountered and documented reusable solutions. This documentation redefined software architecture and established a foundation for ongoing education and practice within the field. Today, understanding this historical context allows developers to appreciate the evolution of their craft and its growing complexity, fostering respect for both the art and science of software engineering.
Technological Considerations
The technological implications of design patterns are significant and multifaceted. As software development practices evolve, the implementation of design patterns facilitates smoother integration with new and emerging technologiesincluding frameworks, libraries, and tools. By embedding established patterns into their work, developers can embrace modern advancements without sacrificing code quality or maintainability, ensuring that their applications remain robust and adaptable in an ever-changing tech landscape. For instance, adopting the MVVM (Model-View-ViewModel) pattern can streamline the integration of reactive programming paradigms, which are increasingly prominent in contemporary app development.
Social Factors
Design patterns also play a critical role in fostering collaboration within development teams. By creating a common language for discussing design and architectural decisions, design patterns enable developers of varied experience levels to communicate effectively. This shared understanding not only enhances teamwork but also promotes mentorship opportunities, whereby more experienced developers can guide less experienced peers in the application and implications of design patterns. Workshops on specific patterns can serve as excellent environments for sharing knowledge and best practices, further ingraining a culture of learning within a team.
Legal and Ethical Aspects
The legal ramifications surrounding software development intersect significantly with design patterns. By utilizing proven paradigms, companies can demonstrate adherence to industry standards, thus mitigating legal liabilities related to software failures or non-compliance. Furthermore, an ethical approach to design encourages developers to consider user privacy and data security implications when implementing these patterns, reinforcing a commitment to responsible and inclusive development practices that respect user rights. As developers prioritize ethical considerations in their work, they contribute to building trust between businesses and their customers, which is increasingly vital in today's digital landscape.
Environmental Perspective
In a time where sustainability is increasingly emphasized, design patterns can facilitate environmentally conscious software design. Patterns promoting resource efficiencysuch as those that minimize energy consumption through optimized algorithmsreflect a broader commitment to ecological considerations. Developers equipped with an understanding of sustainable design principles can create applications that not only fulfill user needs but also contribute positively to the environment. For example, implementing caching design patterns can reduce server load, thus decreasing energy consumption and contributing to a lower carbon footprint.
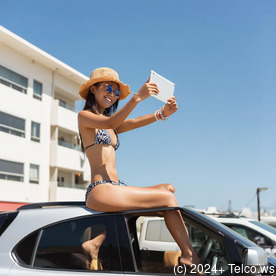
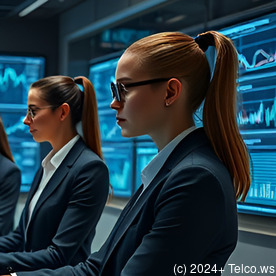
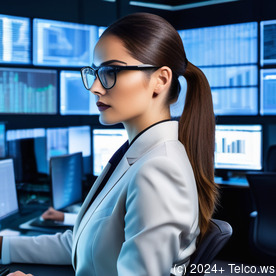
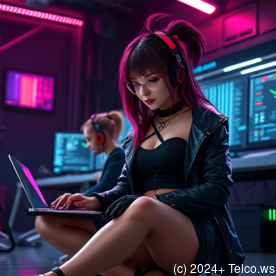
The Technical Landscape of Design Patterns for Swift
Core Concepts and Types of Design Patterns
Within the context of Swift development, design patterns can be broadly categorized into three primary types: Creational, Structural, and Behavioral patterns. Each category addresses specific aspects of system organization and behavior, equipping developers with essential tools to develop structured, organized code effectively:
- Creational Patterns: These patterns deal with object creation mechanisms, providing solutions for managing object instantiation in a controlled manner. Examples of Creational patterns include the Singleton, Factory Method, and Builder patterns. These patterns assist developers in managing object lifecycles and dependencies while avoiding excessive complexity by promoting abstraction in object creation:
- The Singleton pattern ensures a class has only one instance, providing a global point of access to it. This is particularly useful for centralized resource management, such as configurations or network connections.
- The Factory Method pattern defines an interface for creating an object but allows subclasses to alter the type of objects that will be created. This promotes loose coupling, making code easier to maintain and extend.
- The Builder pattern separates the construction of a complex object from its representation, allowing the same construction process to create different representations. This is beneficial for constructing objects with many parameters or multiple variations.
- Structural Patterns: Structural patterns focus on the composition of classes and objects, illustrating how different components can be combined to form larger structures. Examples include the Adapter, Composite, and Decorator patterns, all of which promote enhanced flexibility, code reusability, and easier maintenance:
- The Adapter pattern allows incompatible interfaces to work together, facilitating the integration of new components with existing systems without substantial architectural changes.
- The Composite pattern enables clients to work with individual objects and compositions uniformly. This is particularly useful for representing part-whole hierarchies.
- The Decorator pattern adds new behaviors to objects dynamically without altering their structure. This pattern promotes greater flexibility and adherence to the Open/Closed Principle in object-oriented design.
- Behavioral Patterns: Behavioral patterns emphasize how objects interact and communicate, controlling the flow of communication between them. Key examples include the Observer, Strategy, and Command patterns:
- The Observer pattern enables one object (the subject) to notify multiple observers about state changes, making it ideal for implementing distributed event-handling systems.
- The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This allows clients to choose the appropriate algorithm at runtime, adding flexibility to the application.
- The Command pattern turns requests into objects, allowing for parameterization and queuing of requests. Its useful in implementing undo mechanisms in applications.
Practical Implementation of Patterns
Successfully applying design patterns requires a solid understanding of the challenges they are designed to address. For example, the widely adopted MVC (Model-View-Controller) pattern is a cornerstone of Swift applications, enabling the separation of concerns that simplifies maintenance and enhances scalability. By facilitating this separation, developers can modify the UI, business logic, or data layer independently, which significantly expedites development times and reduces potential regression errors.
Furthermore, utilizing the Observer pattern can greatly benefit applications requiring real-time data updates, such as social media platforms or financial applications. This pattern makes it easier to implement a responsive user interface that reacts to data changes without requiring full-page refreshes or reloads.
Case Studies and Industry Applications
Examining real-world applications of design patterns unveils their invaluable contributions to professional software development environments. For example, an e-commerce platform might effectively utilize the Factory Method pattern to dynamically manage various product typeslike digital downloads and physical goodsstreamlining the process of adding or modifying product offerings without necessitating extensive changes to existing code. This adaptability fosters innovation and responsiveness to market trends, which is crucial in the competitive e-commerce landscape.
Similarly, many applications employ the Singleton pattern for managing database connections. This approach ensures that database interactions are efficient, preventing the overhead associated with establishing multiple connections during concurrent access. By maintaining a single instance of the connection, the application can serve multiple users effectively, ensuring high performance and reliability.
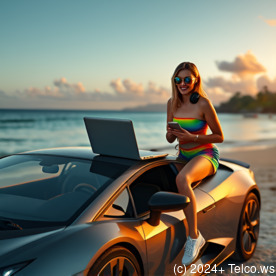
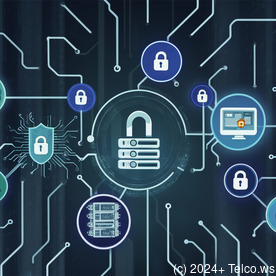
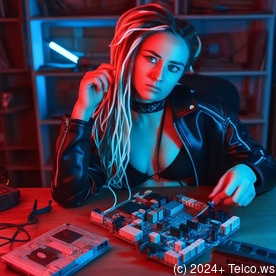
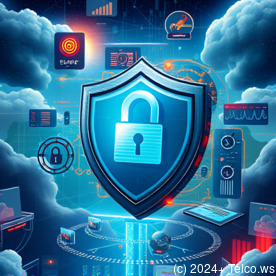
The Case for Investing in Design Patterns Guides
Investing in comprehensive design pattern guides or courses specifically tailored for Swift development holds numerous advantages that can transform a developer's professional trajectory. At telco.ws, we provide in-depth resources that emphasize practical applications and facilitate a deeper understanding of design paradigms. Engaging with our offerings translates into several essential benefits:
- Enhanced Coding Practices: Structured learning methodologies improve coding standards significantly, leading to cleaner, more efficient codebases that are easier to read and maintain. Learning through real-world examples prepares developers to implement best practices effortlessly.
- Expert Insights: Access to industry experts knowledge regarding common pitfalls and effective techniques can aid developers in circumventing costly mistakes, saving both time and financial resources.
- Improved Team Dynamics: A shared vocabulary derived from design patterns enhances collaboration within development teams, simplifying discussions around designs and architectural solutions. This understanding facilitates smoother communication and ensures that teams are aligned on objectives.
- Real-World Applications: Our guides include numerous case studies that demonstrate the practical relevance of design patterns in addressing everyday challenges. By analyzing these real-world examples, developers can develop a more intuitive understanding of how to apply design patterns effectively.
- Preparation for Advanced Concepts: Understanding design patterns provides developers with foundational knowledge crucial for mastering more complex programming concepts and frameworks, such as SwiftUI and Combine.
By equipping themselves with this knowledge, developers can confidently tackle intricate issues and benefit from implementing best practices that lead to high-impact software solutions. The value of hands-on practice in tandem with theoretical knowledge cannot be overstated, and our courses are designed to maximize both aspects.
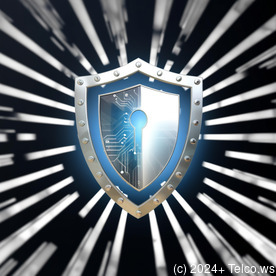
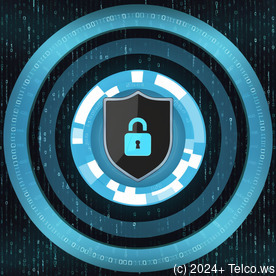
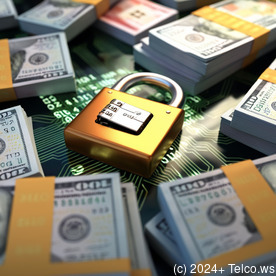
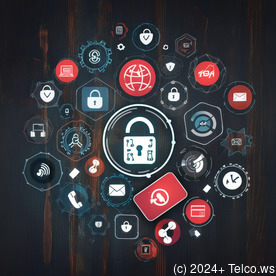
Conclusion: The Future of Design Patterns in Swift Development
Design patterns are poised to continue playing a pivotal role in the evolution of software development practices, particularly within the fast-evolving environment of Swift programming. By integrating design patterns into development workflows, businesses and developers can navigate challenges more effectively, foster innovation, and significantly improve the quality of their software products. As the industry continues to advance, embracing these paradigms will be instrumental in propelling further enhancements in the tech landscape, encouraging a culture of excellence, adaptability, and continuous learning.
In conclusion, the mastery of design patterns not only enhances individual coding abilities but also contributes to the collective intelligence of development teams. It prepares developers to meet the challenges of future technologies while ensuring that their applications are sustainable, efficient, and user-centric.
Unlock Your Potential with Design Patterns Guides!
Are you ready to elevate your Swift development skills to the next level? Our comprehensive design patterns guides and courses are available for $1,199. This investment provides you with powerful strategies and essential tools necessary for achieving success in your programming endeavors. To proceed, please visit our Checkout Gateway and secure your purchase of $1,199 today. After completing your payment, kindly reach out to us via email, phone, or our website with your payment receipt to confirm your enrollment. Thank you for your interest, and we look forward to welcoming you to our vibrant community where learning and growth go hand in hand!